Comment on the output of following C code:
Answer: We are trying to change the value of a const variable. Such code may lead to unknown behaviour in program depending on compiler used. In Dev C++ code compiles with warning and produces output 20 20. Thus value of a constant is being changed through pointers. Such error can be prevented if we make use of pointer to constant to store the address of x instead of a simple integer pointer. If we use int const *p=&x; then *p=20 will produce a compilation error.
Question: What is output of following code snippets
printf("atandon"+1);//P
printf("a%d%d%d"+2,1,2); //Q
Answer & Explanation
P : tandon
+1 removes 1 char from format specifier string.
Q: d12
Question: Explain #ifndef. Is there any alternative to it?
Answer: #ifndef is used to execute a piece of c code if particular macro is not defined.
#ifndef RSA
<code written here will execute only if we have defined constant RSA as #define RSA {some value}>
#endif
We can also use
Question: What is the use of # in macro functions?
Answer: It is used to return the string equivalent of the passed parameter. Consider the following example:-
#define debug(exp) printf(#exp " =%f",exp);
Now debug(x/y); will convert to printf("x/y" " =%f",x/y);
Question: Explain use of ‘##’ in macros.
Answer:
let's say you have
#define dumb_macro(a,b) a ## b
Now if you call the above macro as dumb_macro(hello, world)
It would result in
helloworld
which is not a string, but a symbol(token) and you'll probably end up with an undefined symbol error saying
'helloworld' doesn't exist unless you define it first. To make this legal you first need to declare the symbol like this.
int helloworld;
dumb_macro(hello, world) = 3;
printf ("helloworld = %d\n", helloworld); // <-- would print 'helloworld = 3'
Question: Find output of following code snippet in C++
foo1 b a foo2
Question: What value is returned by cout<<"Hello";
Answer: cout is an object to represent standard output stream. << operator associated with it returns a reference to same standard output stream object. This allows chaining of << operators and thus
cout<<"hello"<<" world";
Question: Which library function will you use to convert a string to equivalent integer?
Answer: int atoi(const char*)
Write a c program to implement your own version of above function?
Answer: Refer
The C Programming Language, second edition,
by Brian Kernighan and Dennis Ritchie
Question: What is fall through? How is it useful?
Answer: Flow of control to following cases after the matching case in absence of break statement after the matching case is known as fall through.
Fall-through allows multiple labels to be associated with a single action.
Question: What is the significance of break statement after default?
Find output of following code:
Explanation:
In P, 10 is substituted in place of * and it indicates putting the value 20 in 10 columns.
By declaring a variable as volatile in C we instruct the compiler that the value of that variable can be changed by means outside the code such as hardware or other program. It is important to note that volatile variables can be constants (declared const) if this code is not allowed to change its value. If we declare a variable as volatile then every time such variable is encountered in the code compiler fetches its value from memory instead of using any optimizations. This ensures that we are reading the correct value of such variable. Volatile memory access is required in case of files which can be read my multiple programs simultaneously.
Question: What is difference between a character stored in a char variable eg. char ch='x' and a simple character constant ‘x’?
Answer:
Look at the following code
size of char constant 4
size of char constant 1
Question: What is the significance of value returned by main in C?
Answer: It tells the environment about the status of program execution. 0 signifies normal termination and non-zero value tells some error in execution. The value returned can be displayed using echo %errorlevel% in Windows and echo $? in UNIX.
Question: In JAVA main function doesn't return any value so how can you tell the environment about the status of program execution?
Question: getchar() is a library function to input a character from console. What data type you should use to store the value returned by this function?
Answer: int !!!
Question: If we have given a pre-processor directive in a c program as "#define <name> <replacement-text>", every occurrence of <name> in program will be replaced by the corresponding <replacement-text>
Answer: False
Question: Find the output of following snippet
#include <stdio.h>
int main(){
const int x=10;
int *p;
p=&x;
*p=20;
printf("%d %d",*p, x);
getch();
return 0;
}
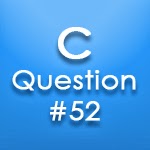
Question: What is output of following code snippets
printf("atandon"+1);//P
printf("a%d%d%d"+2,1,2); //Q
Answer & Explanation
P : tandon
+1 removes 1 char from format specifier string.
Q: d12
+2 will remove 'a' and first % from format specifiers, remaining %d will be replaced by corresponding values => d12
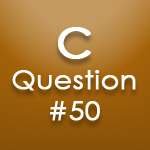
Answer: #ifndef is used to execute a piece of c code if particular macro is not defined.
#ifndef RSA
<code written here will execute only if we have defined constant RSA as #define RSA {some value}>
#endif
We can also use
#if !defined(RSA) instead of #ifndef RSA
Answer: It is used to return the string equivalent of the passed parameter. Consider the following example:-
#define debug(exp) printf(#exp " =%f",exp);
Now debug(x/y); will convert to printf("x/y" " =%f",x/y);
This will print: x/y =<value of x/y in float>
Answer:
let's say you have
#define dumb_macro(a,b) a ## b
Now if you call the above macro as dumb_macro(hello, world)
It would result in
helloworld
which is not a string, but a symbol(token) and you'll probably end up with an undefined symbol error saying
'helloworld' doesn't exist unless you define it first. To make this legal you first need to declare the symbol like this.
int helloworld;
dumb_macro(hello, world) = 3;
printf ("helloworld = %d\n", helloworld); // <-- would print 'helloworld = 3'
## is called token passing argument since it is used to create tokens through passed arguments
#include <iostream> #include <conio.h> using namespace std; int foo1(){ cout<<"foo1 "; return 1; } int foo2(void* a, void* b){ cout<<"foo2 "; return 2; } int main(){ int x=~foo1()&~foo2(cout<<"a ",cout<<"b ");//foo1 b a foo2 getch(); }Answer:
foo1 b a foo2
Explanation: << operator associated with cout object returns a reference to itself this can be accepted by void* (generic pointer) ~ is negation operator. The output depends on order of execution of expression and order in which parameters are passed in function.
Answer: cout is an object to represent standard output stream. << operator associated with it returns a reference to same standard output stream object. This allows chaining of << operators and thus
cout<<"hello"<<" world";
is same as cout<<"hello world";
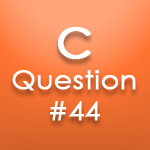
Answer: int atoi(const char*)
Write a c program to implement your own version of above function?
Answer: Refer
The C Programming Language, second edition,
by Brian Kernighan and Dennis Ritchie
Answer: Flow of control to following cases after the matching case in absence of break statement after the matching case is known as fall through.
Fall-through allows multiple labels to be associated with a single action.
Question: What is the significance of break statement after default?
Answer: Fall through can occur after default too. If default is not the last label and there are other labels after it without any break in between. Therefore as a defensive programming you may add a break after default too although it is logically unnecessary.
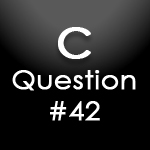
#include <stdio.h> main() { printf ("%*d\n", 10, 20); // P printf ("%*d\n", 20, 10); // Q }
Explanation:
In P, 10 is substituted in place of * and it indicates putting the value 20 in 10 columns.
In Q, 20 is substituted for * and it indicates putting the value 10 in 20 columns.
Question: What is the use of volatile keyword in C Language?
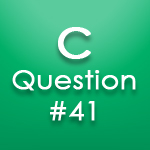
Answer:
Look at the following code
main() { char b = 'c'; printf("size of char constant %d\n", (int)sizeof('a')); printf("size of char variable %d",(int)sizeof(b)); }Output:
size of char constant 4
size of char constant 1
Explanation: According to the ANSI/ISO 99 C standard, the expression 'a' starts with type int. If you have a function void f(int) and a variable char c, then f(c) will perform integral promotion, but f('a') won't because the type of 'a' is already int.
Answer: It tells the environment about the status of program execution. 0 signifies normal termination and non-zero value tells some error in execution. The value returned can be displayed using echo %errorlevel% in Windows and echo $? in UNIX.
Question: In JAVA main function doesn't return any value so how can you tell the environment about the status of program execution?
Answer: We can use System.exit(0); or System.exit(<non-zero error code as integer>);
Question: getchar() is a library function to input a character from console. What data type you should use to store the value returned by this function?
Answer: int !!!
Explanation: EOF is an integer constant defined in stdio.h while reading characters using getchar() EOF is returned instead of ASCII value of the character when no more characters are available. Therefore we must read the input from getchar() in an integer and not char directly so that the variable is big enough to read the EOF value.
Answer: False
Explanation: Replacements won't be made when <name> occurs as part of other name Eg. <prefix-name> also replacements won't be made if <name> occurs inside quotes.
Question: What is the output for following code snippet?
float d=22.44;
printf("%-8.1f",d);
printf("%-8.1f",d);
Answer: %X.Yf prints a float value right justified in X columns up to 1 digit after decimal. Minus can be used in format specifier to make the text left justified. Thus the code tells to print 22.44 as 22.4 left justified in a column whose width is eight.
Question: What are the input and output functions defined in C language?
Answer: There is no input or output function defined in c language. printf & scanf are parts of standard input output library that is normally accessible to a c program. The behaviour of standard input output functions are defined in ANSI standard and its properties should be same in any compiler that follows the standards.
#include <stdio.h> int main(){ int c,f=100; c=5/9*(f-32); //A printf("%d\n",c); c=5.0/9.0*(f-32);//B printf("%d\n",c); c=5*(f-32)/9;//C printf("%d\n",c); getch(); }
Output:
0
37
37
Explanation: In c automatic truncation to integer is done for int data type variables. On using float/double constant same can be prevented with the help of automatic type promotion.
No comments:
Post a Comment